Welcome to [GHB] - GAmEhAcKbAsTaRdS Forum
- Start new topics and reply to others
- Subscribe to topics and forums to get email updates
- Get your own profile page and make new friends
- Send personal messages to other members.
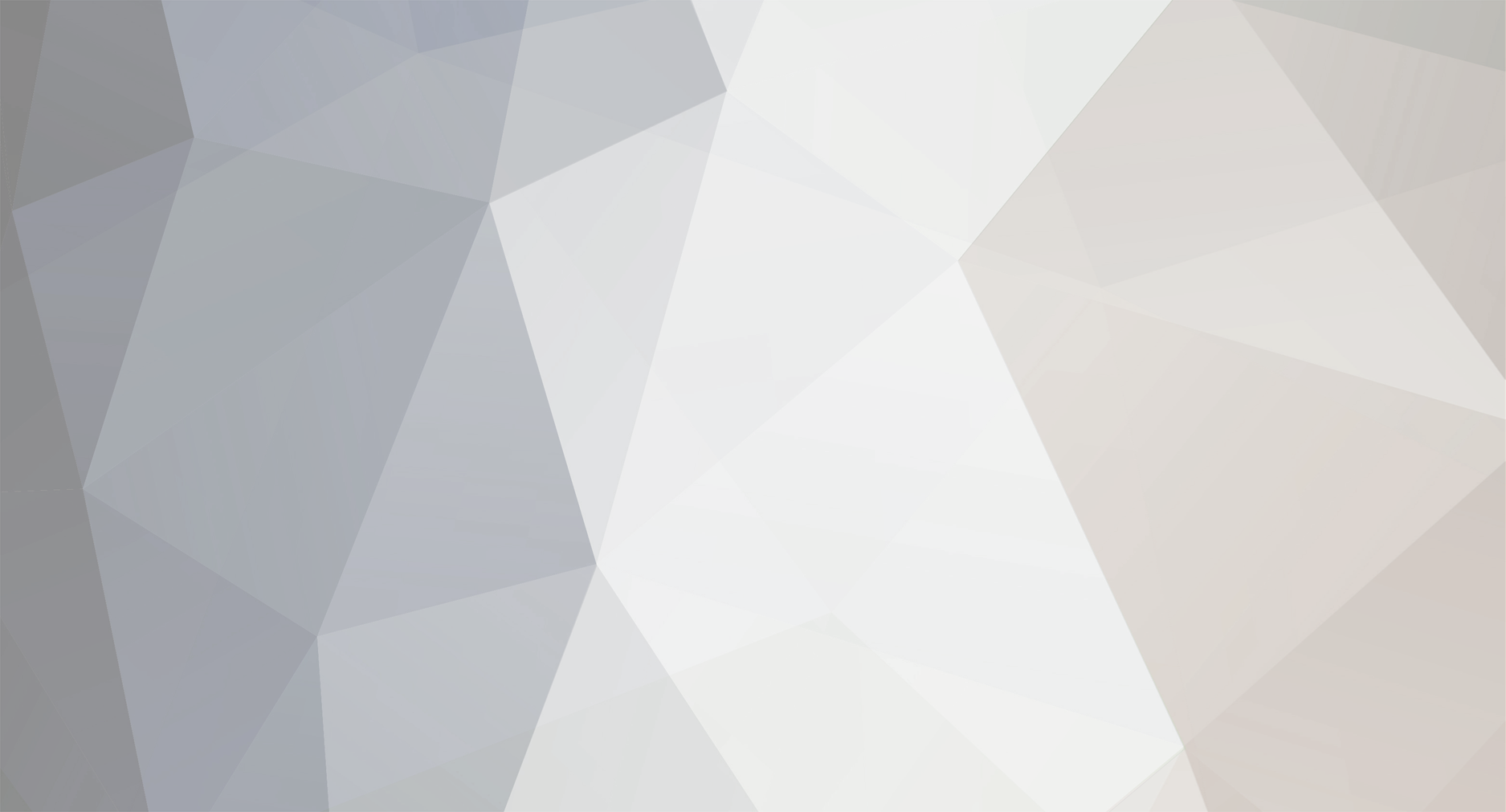
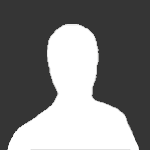
a1fahrrad
-
Posts
132 -
Joined
-
Last visited
Content Type
Profiles
Forums
Calendar
Downloads
Store
Posts posted by a1fahrrad
-
-
picco
-
float -> 4
-
-
As example I'm using Stamina from WarRock
#define Playerpointer 0xAddy #define StaminaOff 0xOffset int Stamina; while(1) { float bsp1; float bsp2; DWORD Stamina2 = *(DWORD*)(Playerpointer + StaminaOff ); if(Stamina == 1) { Stamina2 = bsp1; if(bsp1>= 35 ) { *(float*)(Stamina 2) = (float)35; } } }
This should work, the code isn't 100 % correct -> written from my memory.
-
GetAsyncKeyState
learn C++ and don't spam -.-
-
i made this...
I don't know if there is a Tutorial...
It's not hard to code...
& i stopped coding and delete my projekts -> i can't share it or something else
-
if(GetAsynckEyState(VK_INSERT))
{
DrawRectangle(X, Y, width, high, size, Color, Device );
}
@CrazyMike
I made this without Source Code or something, you can do it with a little bit mathematik knowledge
-
I was a bit creativ
The Result:
-
The starterkid is patched for the most new online games.
@Topic:
Use a normal C++ Compiler and include some files from the SDK
-
Hotkeys:
If(GetAsyncKeyState(Key) ) {//speed code }
-
@ledzepfan1: This isn't the Playerpointer lol..., Serverpointer
I think this will work
#define ADR_Playerpointer 0x1522078 #define OFF_NFD 0x280 void NFD( ) { DWORD dwPlayerpointer = *(DWORD*)ADR_Playerpointer; if(dwPlayerpointer != 0) { *(float*)(dwPlayerpointer+ OFS_NFD) = -20000; } }
-
You need 2 Funktions:
BOOL WritePrivateProfileInt(LPCTSTR lpAppName, LPCTSTR lpKeyName, int nInteger, LPCTSTR lpFileName) { TCHAR lpString[ 1024 ]; wsprintf( lpString, "%d", nInteger ); return WritePrivateProfileString( lpAppName, lpKeyName, lpString, lpFileName ); }
UINT WINAPI GetPrivateProfileInt( __in LPCTSTR lpAppName, __in LPCTSTR lpKeyName, __in INT nDefault, __in LPCTSTR lpFileName );
How to Use:
void Load() { Chams = GetPrivateProfileIntA("D3D", "Chams", Chams, "Bsp.txt"); } void Save() { WritePrivateProfileInt("D3D", "Chams", Chams, Bsp.txt")); }
Credits:
To the one who made the WritePrivatProfileInt Funktion
MSDN
a1fahrrad (4 writing the Tutorial)
-
The DrawBox Funktion
void DrawBox( LPDIRECT3DDEVICE9 pDevice , int x, int y, int w, int h, DWORD Color ) { D3DRECT rec; rec.x1 = x; rec.x2 = x + w; rec.y1 = y; rec.y2 = y + h; pDevice->Clear( 1, &rec, D3DCLEAR_TARGET, Color, 0, 0 ); }
The Rectangle Funktion:
void DrawRectangle(int x, int y, int w, int h, int s, DWORD Color, LPDIRECT3DDEVICE9 pDevice) { DrawBox(pDevice, x, y, w, s, Color ); DrawBox(pDevice, x, y, s, h, Color ); DrawBox(pDevice, (x+w), y, s, h, Color ); DrawBox(pDevice, x, (y+h), w+s, s, Color ); }
How To use:
DrawRectangle(X, Y, width, high, size, Color, Device );
Credits:
To the one who made the FillRGB Funktion.
a1fahrrad (Rectangle Funktion)
-
Write it in a txt File. I won't give you a Source. Ask Google
-
lol?
Undeclared -> max_component_length
I think you have to learn to read & learn C++ a bit more.
DWORD max_component_length;
-
Reset your Fonts then it won't crash.
-
dword_150FFA0 is the Playerpointer, now you have to get the sig...
-
First you need a Draw Funktion, i'm using Hans211 DrawBox Funktion
void DrawBox(IDirect3DDevice8 *pDevice, int x, int y, int w, int h, D3DCOLOR col) { struct QuadVertex { float x,y,z,rhw; DWORD dwColor; }; IDirect3DVertexBuffer8 *pVertexBuffer = NULL; QuadVertex qV[4]; BYTE *pVertexData = NULL; if (pDevice->CreateVertexBuffer((4*sizeof(QuadVertex)),(D3DUSAGE_WRITEONLY|D3DUSAGE_DYNAMIC),(D3DFVF_XYZRHW|D3DFVF_DIFFUSE),D3DPOOL_DEFAULT,&pVertexBuffer)<0) return; pDevice->SetRenderState(D3DRS_ZENABLE , FALSE); pDevice->SetRenderState(D3DRS_CULLMODE, D3DCULL_NONE); pDevice->SetRenderState(D3DRS_LIGHTING, FALSE); pVertexBuffer->Lock(0,0,&pVertexData,D3DLOCK_NOSYSLOCK | D3DLOCK_DISCARD); if(pVertexData) { qV[0].dwColor = qV[1].dwColor = qV[2].dwColor = qV[3].dwColor = col; qV[0].z = qV[1].z = qV[2].z = qV[3].z = 0.0f; qV[0].rhw = qV[1].rhw = qV[2].rhw = qV[3].rhw = 0.0f; qV[0].x = (float)x; qV[0].y = (float)(y + h); qV[1].x = (float)x; qV[1].y = (float)y; qV[2].x = (float)(x + w); qV[2].y = (float)(y + h); qV[3].x = (float)(x + w); qV[3].y = (float)y; memcpy(pVertexData,qV,sizeof(QuadVertex)*4); pVertexBuffer->Unlock(); pVertexData = NULL; pDevice->SetStreamSource(0,pVertexBuffer, sizeof(QuadVertex)); pDevice->SetVertexShader( D3DFVF_XYZRHW | D3DFVF_DIFFUSE ); pDevice->DrawPrimitive( D3DPT_TRIANGLESTRIP, 0, 2 ); } if (pVertexBuffer) { pVertexBuffer->Release(); pVertexBuffer=NULL; } pDevice->SetRenderState(D3DRS_LIGHTING, true); }
Here is the Mouse Funktion:
void DrawCursor1(int x, int y, DWORD Color1, DWORD Color2, LPDIRECT3DDEVICE8 pDevice ) { //Credits to a1fahrrad DrawBox(pDevice, x, y, 1, 1, Color1 ); DrawBox(pDevice, x, y, 1, 12, Color1 ); DrawBox(pDevice, x+1, y+11, 1, 1, Color1 ); DrawBox(pDevice, x+2, y+10, 1, 1, Color1 ); DrawBox(pDevice, x+3, y+9, 1, 1, Color1 ); DrawBox(pDevice, x+4, y+10, 1, 2, Color1 ); DrawBox(pDevice, x+5, y+12, 1, 2, Color1 ); DrawBox(pDevice, x+6, y+14, 2, 1, Color1 ); DrawBox(pDevice, x+7, y+10, 1, 2, Color1 ); DrawBox(pDevice, x+6, y+9 , 1, 1, Color1 ); DrawBox(pDevice, x+8, y+12, 1, 2, Color1 ); DrawBox(pDevice, x+1, y, 1, 1, Color1 ); DrawBox(pDevice, x+2, y+1, 1, 1, Color1 ); DrawBox(pDevice, x+3, y+2, 1, 1, Color1 ); DrawBox(pDevice, x+4, y+3, 1, 1, Color1 ); DrawBox(pDevice, x+5, y+4, 1, 1, Color1 ); DrawBox(pDevice, x+6, y+5, 1, 1, Color1 ); DrawBox(pDevice, x+7, y+6, 1, 1, Color1 ); DrawBox(pDevice, x+8, y+7, 1, 1, Color1 ); DrawBox(pDevice, x+8, y+8, 1, 1, Color1 ); DrawBox(pDevice, x+7, y+8, 1, 1, Color1 ); DrawBox(pDevice, x+6, y+8, 1, 1, Color1 ); DrawBox(pDevice, x+1, y+1, 1, 1, Color2 ); DrawBox(pDevice, x+1, y+2, 2, 1, Color2 ); DrawBox(pDevice, x+1, y+3, 3, 1, Color2 ); DrawBox(pDevice, x+1, y+4, 4, 1, Color2 ); DrawBox(pDevice, x+1, y+5, 5, 1, Color2 ); DrawBox(pDevice, x+1, y+6, 6, 1, Color2 ); DrawBox(pDevice, x+1, y+7, 7, 1, Color2 ); DrawBox(pDevice, x+1, y+8, 5, 1, Color2 ); DrawBox(pDevice, x+1, y+9, 2, 1, Color2 ); DrawBox(pDevice, x+1, y+10, 1, 1, Color2 ); DrawBox(pDevice, x+4, y+9, 2, 1, Color2 ); DrawBox(pDevice, x+5, y+10, 2, 2, Color2 ); DrawBox(pDevice, x+6, y+12, 2, 2, Color2 ); }
Use GetCursorPos( ); to get the Position of the Cursor!
Screen
-
The normal Addies like Playerpointer are Big Endians
Playerpointer(Big Endian): 01 50 FF A0 -> Little Endian: A0 FF 50 01
Now search with Olly with Binary Search and get the Sig.
-
-
@ColdHearth: The Starterkid isn't patched, only the method to get the Direkt 3D DevicePointer is in WarRock patched.
@Topic:
Show if your SDK is right included.
-
Search the Addy as little Endian with the Binary Search.
-
nice work
-
D3D8.dll hooken -> Gordon hat mal ein TuT dafür gemacht
Eigene Endscene erstellen -> Fonts reseten.
Drawen.
WarRock Bypass Source Code
in C and C++
Posted
rep